SWT中使用JFreeChart(无需SWT_AWT)
好像从1.03开始Jfc就已经提供了在SWT中使用JFC的专用包和类,只是没有人写这些东西而已~今天我就贴一些Demo,以后再也不用SWT_AWT了~
更重要的是SWT_AWT主要是将AWT嵌入SWT中,而ChartComposite则是将chart对象直接转换为SWT中的东东,连右键都是SWT的了~
声明:
永久性关闭本Blog,内容已经迁往 www.lign.name 全新的空间,全新的内容,敬请关注!
1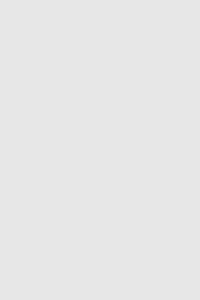
/**//* ===========================================================
2
* JFreeChart : a free chart library for the Java(tm) platform
3
* ===========================================================
4
*
5
* (C) Copyright 2000-2007, by Object Refinery Limited and Contributors.
6
*
7
* Project Info: http://www.jfree.org/jfreechart/index.html
8
*
9
* This library is free software; you can redistribute it and/or modify it
10
* under the terms of the GNU Lesser General Public License as published by
11
* the Free Software Foundation; either version 2.1 of the License, or
12
* (at your option) any later version.
13
*
14
* This library is distributed in the hope that it will be useful, but
15
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
16
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
17
* License for more details.
18
*
19
* You should have received a copy of the GNU Lesser General Public
20
* License along with this library; if not, write to the Free Software
21
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301,
22
* USA.
23
*
24
* [Java is a trademark or registered trademark of Sun Microsystems, Inc.
25
* in the United States and other countries.]
26
*
27
* ---------------------
28
* SWTBarChartDemo1.java
29
* ---------------------
30
* (C) Copyright 2006, 2007, by Object Refinery Limited and Contributors.
31
*
32
* Original Author: David Gilbert (for Object Refinery Limited);
33
* Contributor(s):
34
*
35
* Changes
36
* -------
37
* 23-Aug-2006 : New class (DG);
38
*
39
*/
40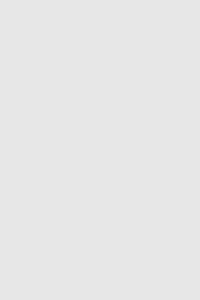
41
package org.jfree.experimental.chart.swt.demo;
42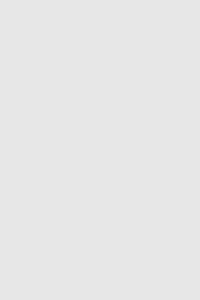
43
import java.awt.Color;
44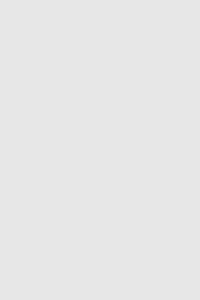
45
import org.eclipse.swt.SWT;
46
import org.eclipse.swt.layout.FillLayout;
47
import org.eclipse.swt.widgets.Display;
48
import org.eclipse.swt.widgets.Shell;
49
import org.jfree.chart.ChartFactory;
50
import org.jfree.chart.JFreeChart;
51
import org.jfree.chart.axis.CategoryAxis;
52
import org.jfree.chart.axis.CategoryLabelPositions;
53
import org.jfree.chart.axis.NumberAxis;
54
import org.jfree.chart.plot.CategoryPlot;
55
import org.jfree.chart.plot.PlotOrientation;
56
import org.jfree.chart.renderer.category.BarRenderer;
57
import org.jfree.data.category.CategoryDataset;
58
import org.jfree.data.category.DefaultCategoryDataset;
59
import org.jfree.experimental.chart.swt.ChartComposite;
60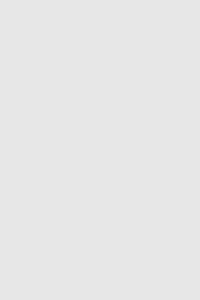
61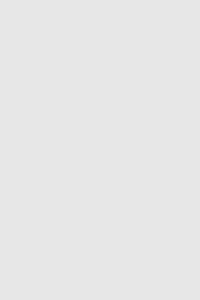
/** *//**
62
* An SWT demo.
63
*/
64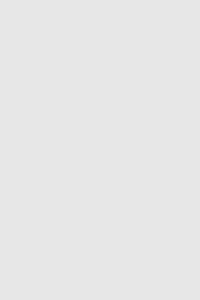
public class SWTBarChartDemo1
{
65
66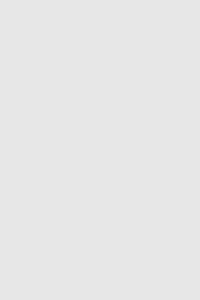
/** *//**
67
* Returns a sample dataset.
68
*
69
* @return The dataset.
70
*/
71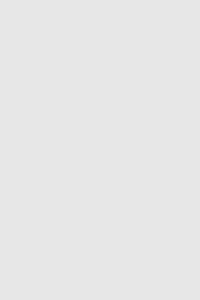
private static CategoryDataset createDataset()
{
72
73
// row keys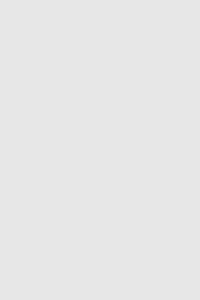
74
String series1 = "First";
75
String series2 = "Second";
76
String series3 = "Third";
77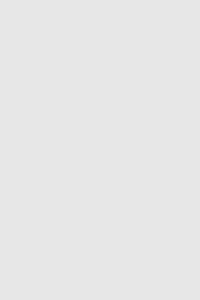
78
// column keys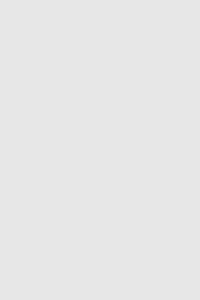
79
String category1 = "Category 1";
80
String category2 = "Category 2";
81
String category3 = "Category 3";
82
String category4 = "Category 4";
83
String category5 = "Category 5";
84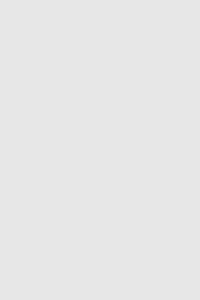
85
// create the dataset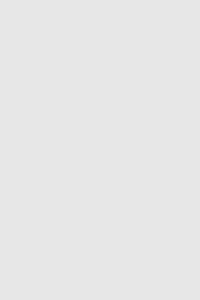
86
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
87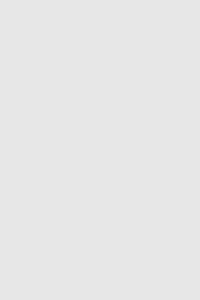
88
dataset.addValue(1.0, series1, category1);
89
dataset.addValue(4.0, series1, category2);
90
dataset.addValue(3.0, series1, category3);
91
dataset.addValue(5.0, series1, category4);
92
dataset.addValue(5.0, series1, category5);
93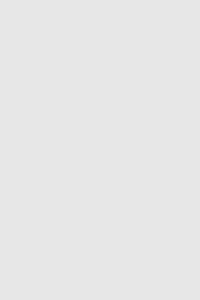
94
dataset.addValue(5.0, series2, category1);
95
dataset.addValue(7.0, series2, category2);
96
dataset.addValue(6.0, series2, category3);
97
dataset.addValue(8.0, series2, category4);
98
dataset.addValue(4.0, series2, category5);
99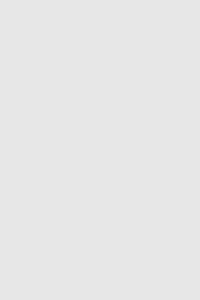
100
dataset.addValue(4.0, series3, category1);
101
dataset.addValue(3.0, series3, category2);
102
dataset.addValue(2.0, series3, category3);
103
dataset.addValue(3.0, series3, category4);
104
dataset.addValue(6.0, series3, category5);
105
106
return dataset;
107
108
}
109
110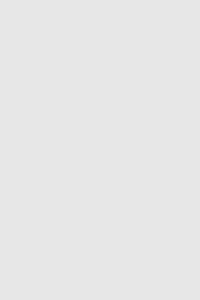
/** *//**
111
* Creates a sample chart.
112
*
113
* @param dataset the dataset.
114
*
115
* @return The chart.
116
*/
117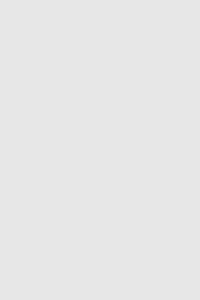
private static JFreeChart createChart(CategoryDataset dataset)
{
118
119
// create the chart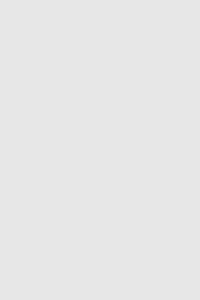
120
JFreeChart chart = ChartFactory.createBarChart(
121
"Bar Chart Demo", // chart title
122
"Category", // domain axis label
123
"Value", // range axis label
124
dataset, // data
125
PlotOrientation.VERTICAL, // orientation
126
true, // include legend
127
true, // tooltips?
128
false // URLs?
129
);
130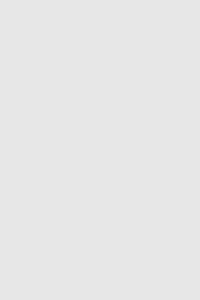
131
// NOW DO SOME OPTIONAL CUSTOMISATION OF THE CHART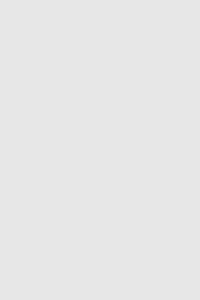
132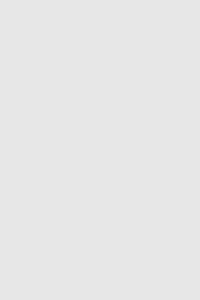
133
// set the background color for the chart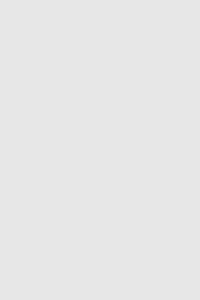
134
chart.setBackgroundPaint(Color.white);
135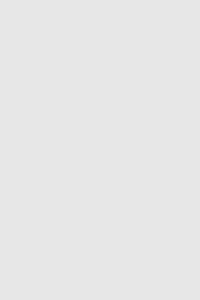
136
// get a reference to the plot for further customisation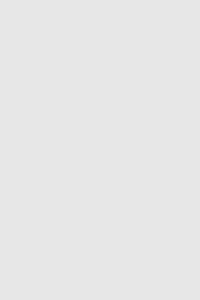
137
CategoryPlot plot = (CategoryPlot) chart.getPlot();
138
plot.setBackgroundPaint(Color.lightGray);
139
plot.setDomainGridlinePaint(Color.white);
140
plot.setDomainGridlinesVisible(true);
141
plot.setRangeGridlinePaint(Color.white);
142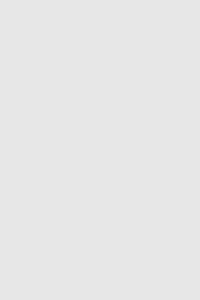
143
// set the range axis to display integers only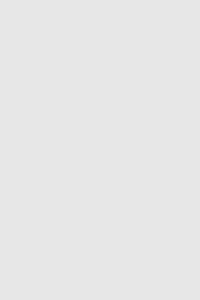
144
final NumberAxis rangeAxis = (NumberAxis) plot.getRangeAxis();
145
rangeAxis.setStandardTickUnits(NumberAxis.createIntegerTickUnits());
146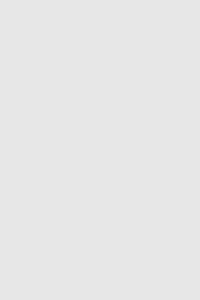
147
// disable bar outlines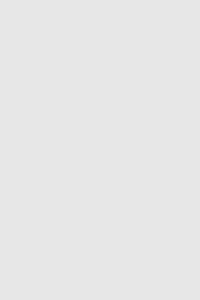
148
BarRenderer renderer = (BarRenderer) plot.getRenderer();
149
renderer.setDrawBarOutline(false);
150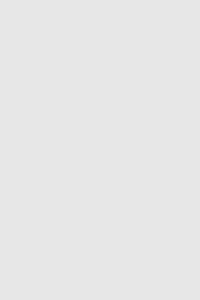
151
CategoryAxis domainAxis = plot.getDomainAxis();
152
domainAxis.setCategoryLabelPositions(
153
CategoryLabelPositions.createUpRotationLabelPositions(Math.PI / 6.0)
154
);
155
// OPTIONAL CUSTOMISATION COMPLETED.
156
157
return chart;
158
159
}
160
161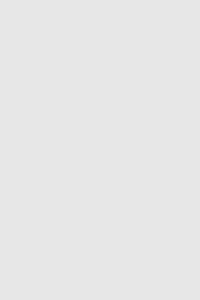
/** *//**
162
* Starting point for the demonstration application.
163
*
164
* @param args ignored.
165
*/
166
public static void main( String[] args )
167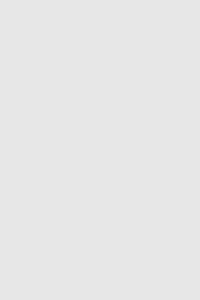
{
168
JFreeChart chart = createChart(createDataset());
169
Display display = new Display();
170
Shell shell = new Shell(display);
171
shell.setSize(600, 300);
172
shell.setLayout(new FillLayout());
173
shell.setText("Test for jfreechart running with SWT");
174
final ChartComposite frame = new ChartComposite(shell, SWT.NONE, chart,
175
true);
176
frame.pack();
177
shell.open();
178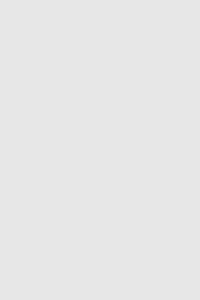
while (!shell.isDisposed())
{
179
if (!display.readAndDispatch())
180
display.sleep();
181
}
182
}
183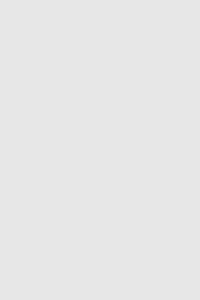
184
}
185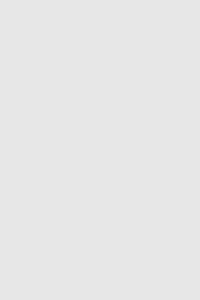
186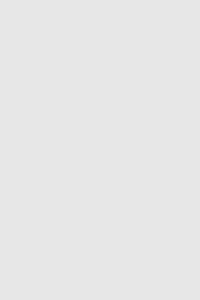
187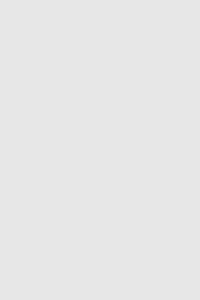
这个是JFC里面自带的一个例子,例子里面使用了一个ChartComposite来放置chart对象~这样对使用者来说更加方便了,其实JFC的SWT包下还有好几个很好用的类,只是没有文档而已~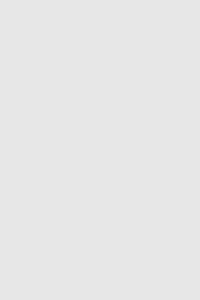
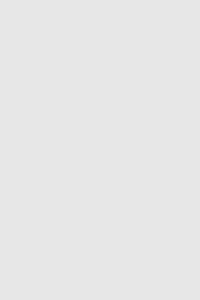
2
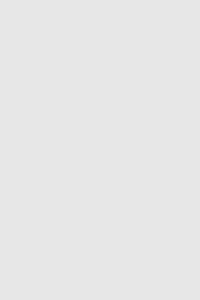
3
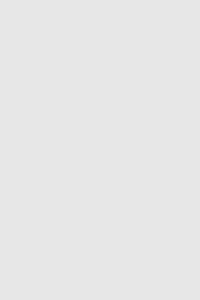
4
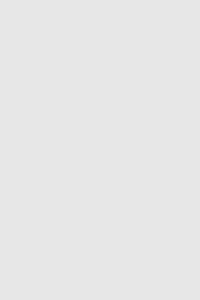
5
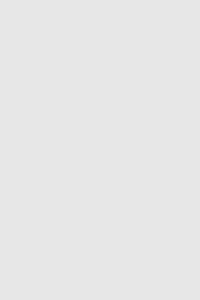
6
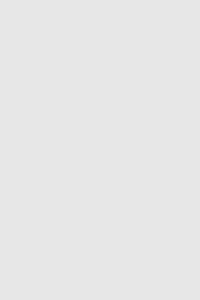
7
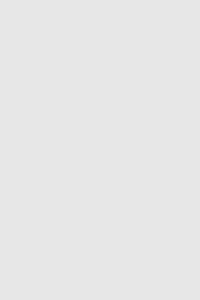
8
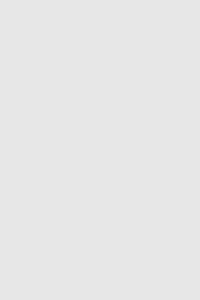
9
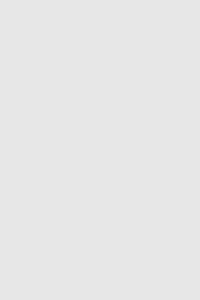
10
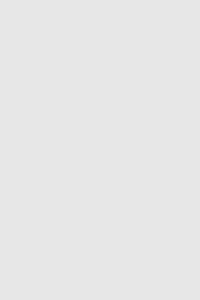
11
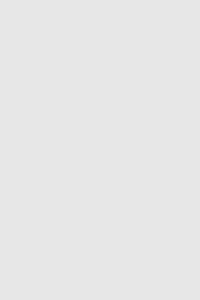
12
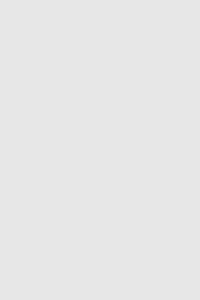
13
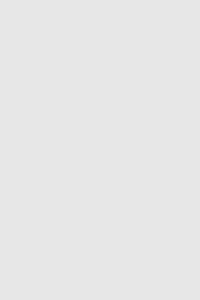
14
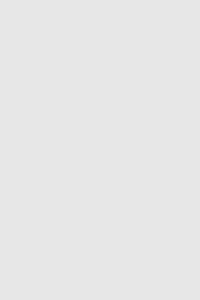
15
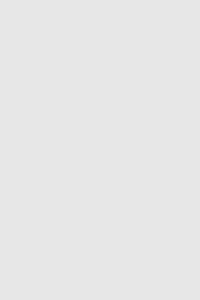
16
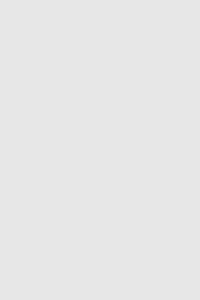
17
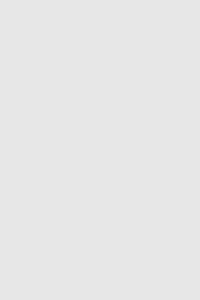
18
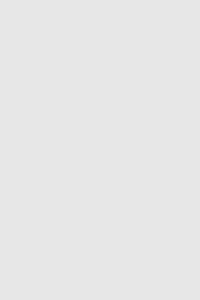
19
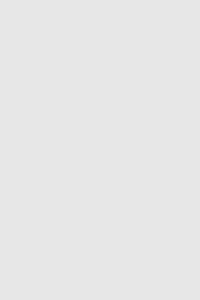
20
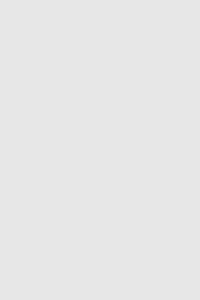
21
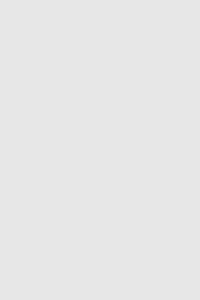
22
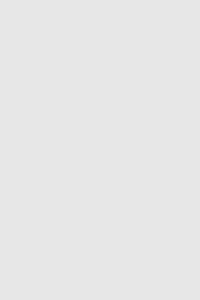
23
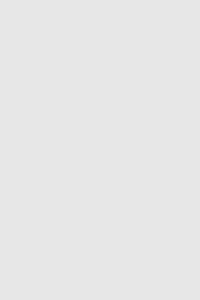
24
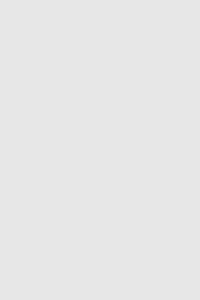
25
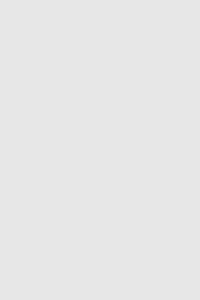
26
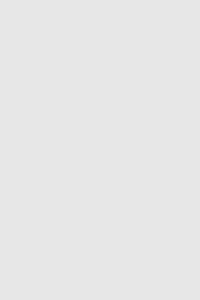
27
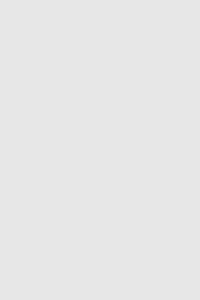
28
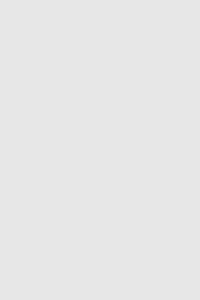
29
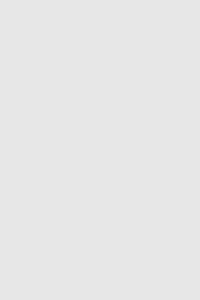
30
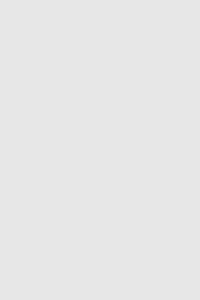
31
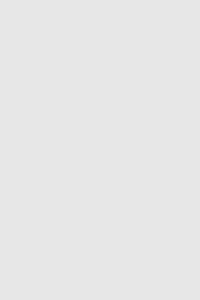
32
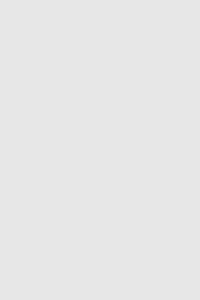
33
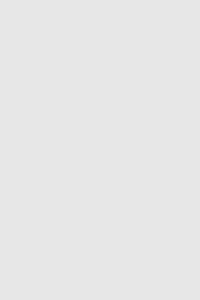
34
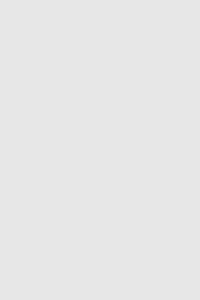
35
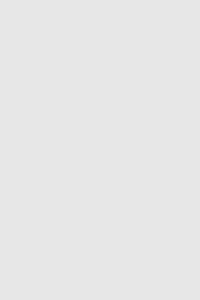
36
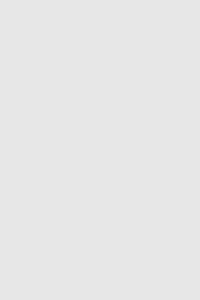
37
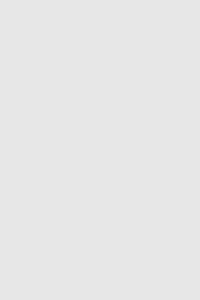
38
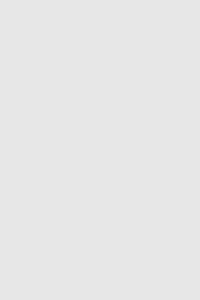
39
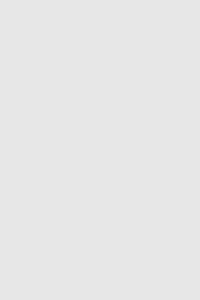
40
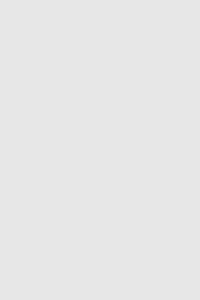
41
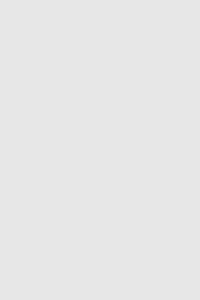
42
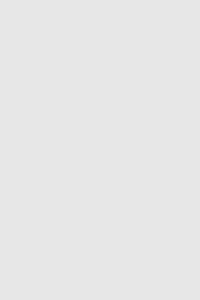
43
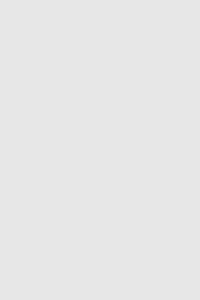
44
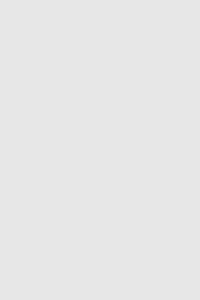
45
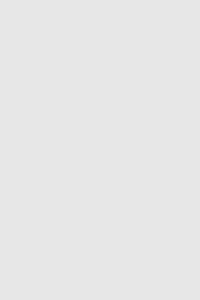
46
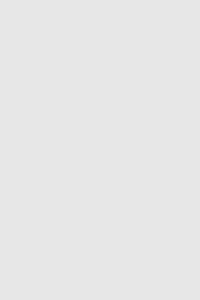
47
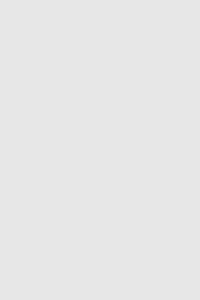
48
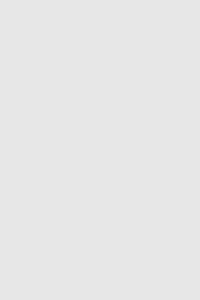
49
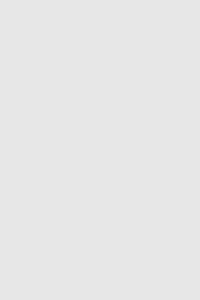
50
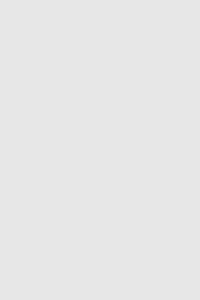
51
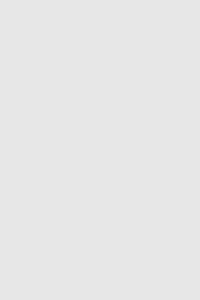
52
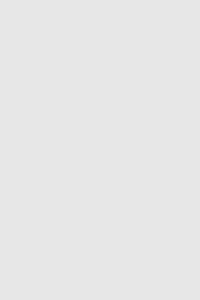
53
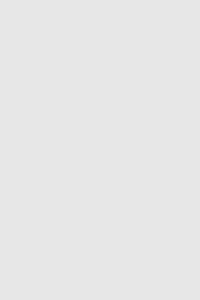
54
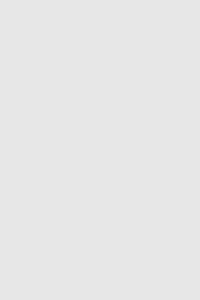
55
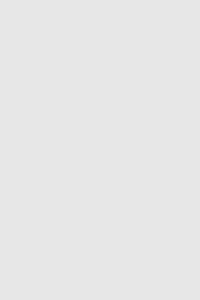
56
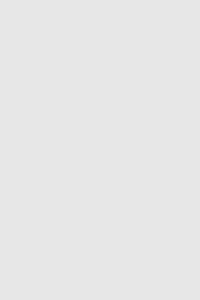
57
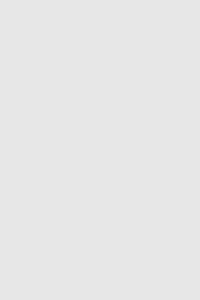
58
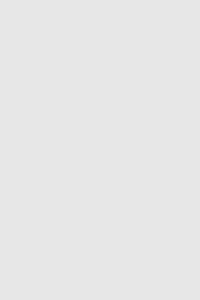
59
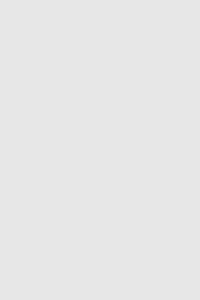
60
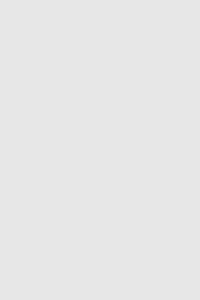
61
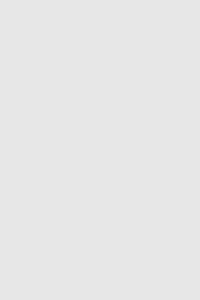
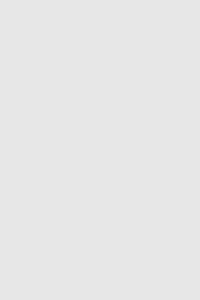
62
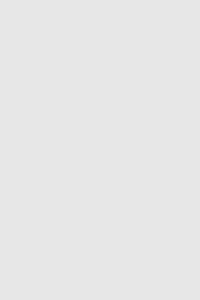
63
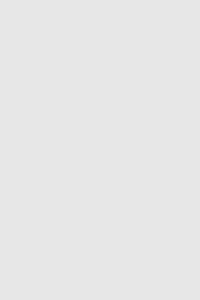
64
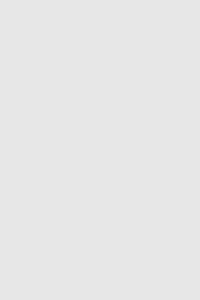
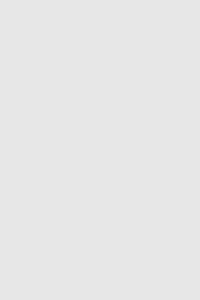
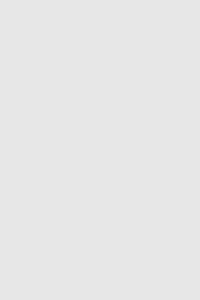
65
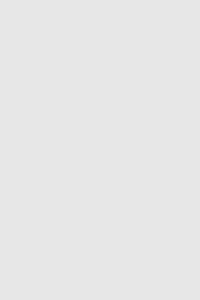
66
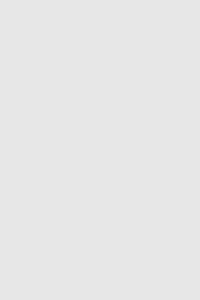
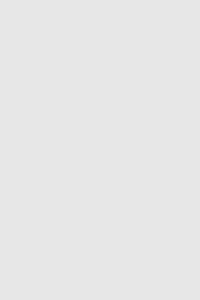
67
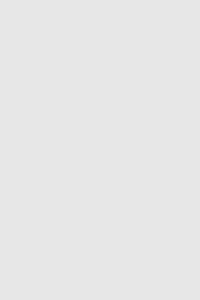
68
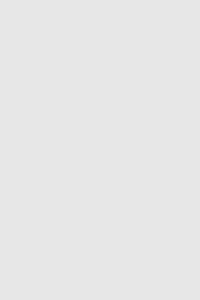
69
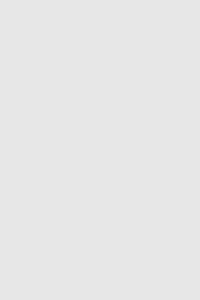
70
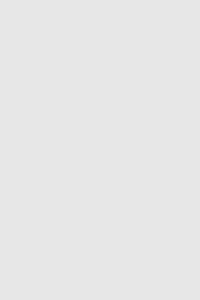
71
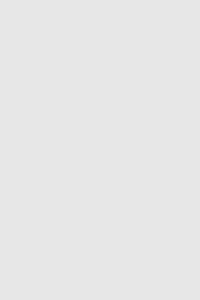
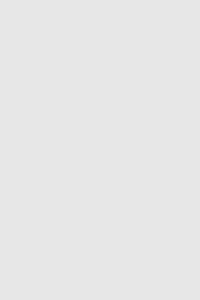
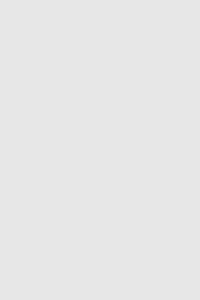
72
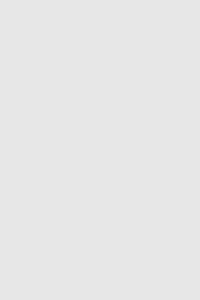
73
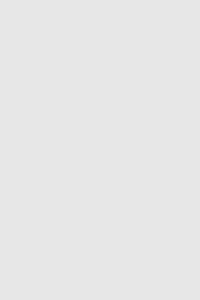
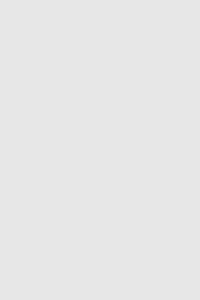
74
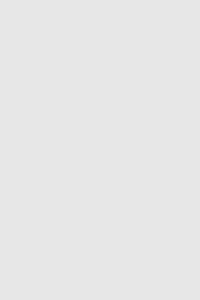
75
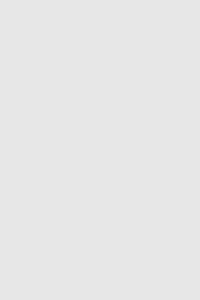
76
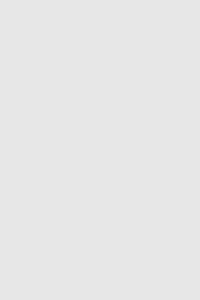
77
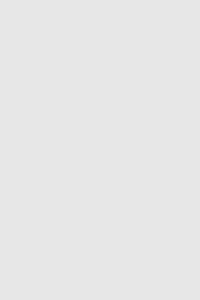
78
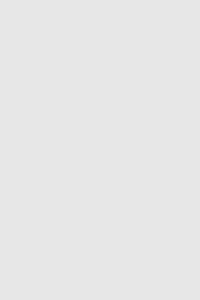
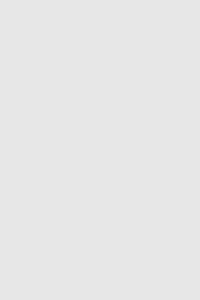
79
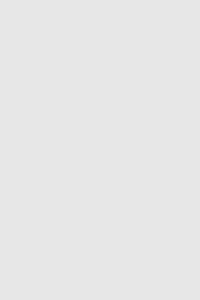
80
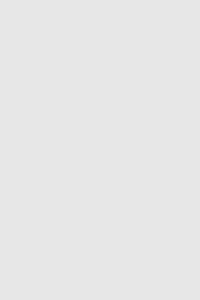
81
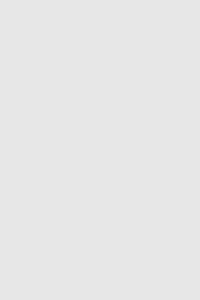
82
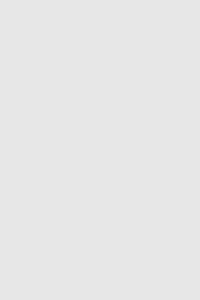
83
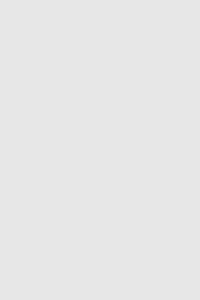
84
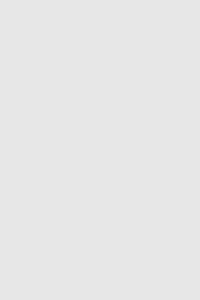
85
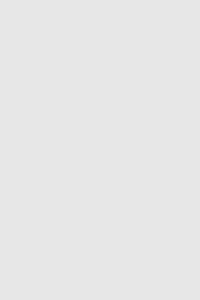
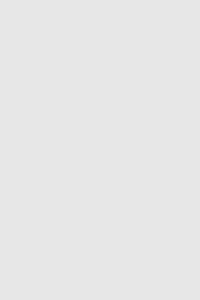
86
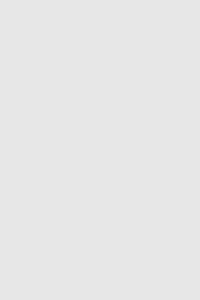
87
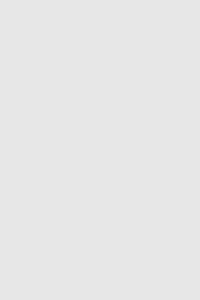
88
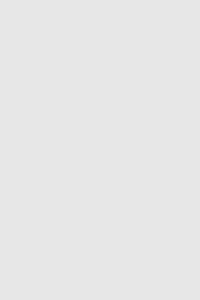
89
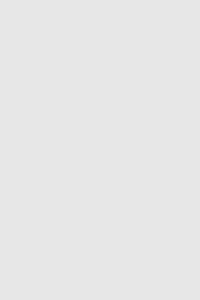
90
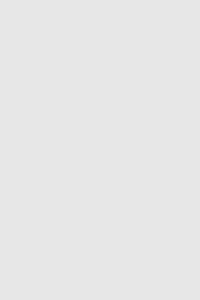
91
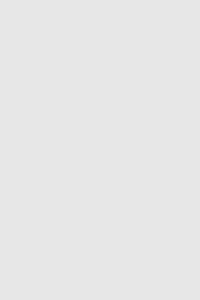
92
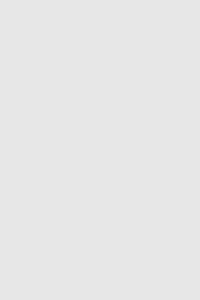
93
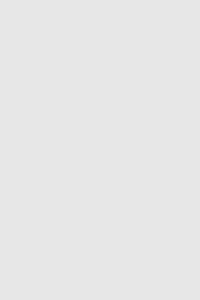
94
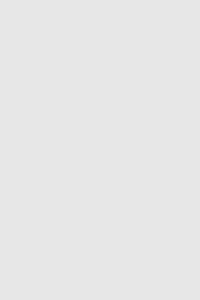
95
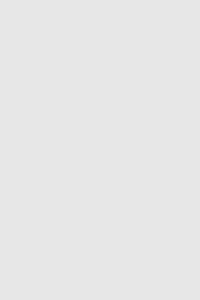
96
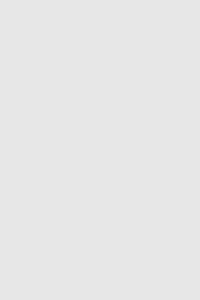
97
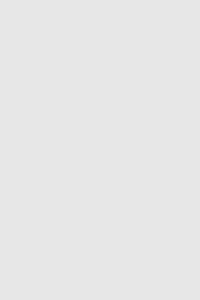
98
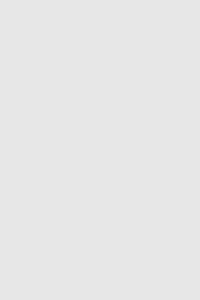
99
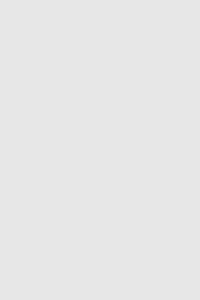
100
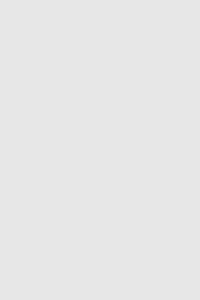
101
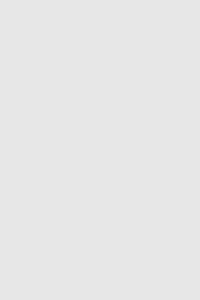
102
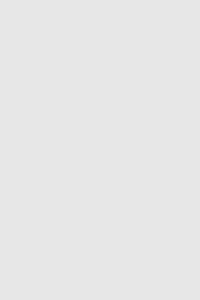
103
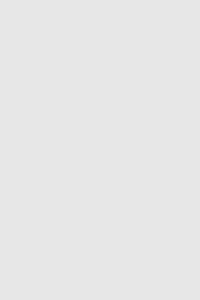
104
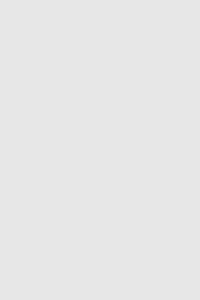
105
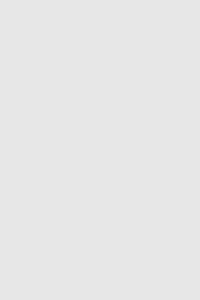
106
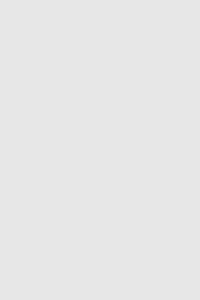
107
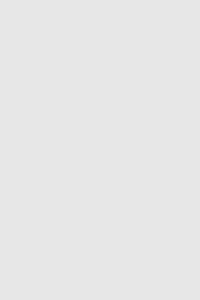
108
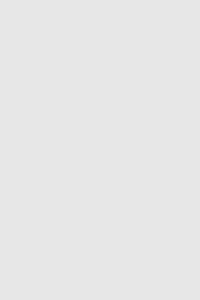
109
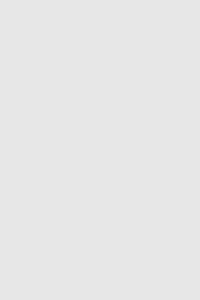
110
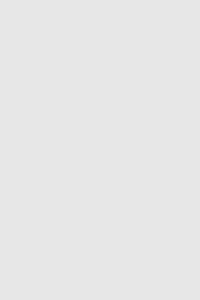
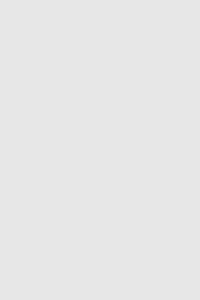
111
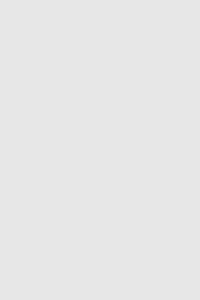
112
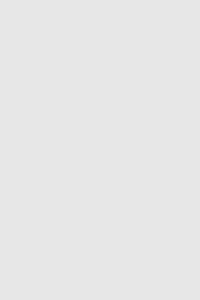
113
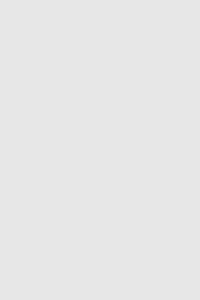
114
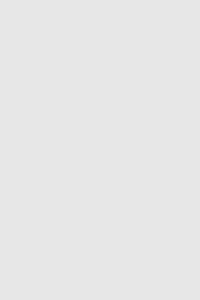
115
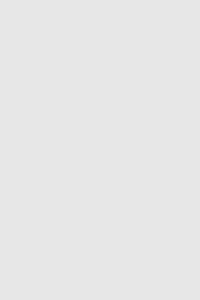
116
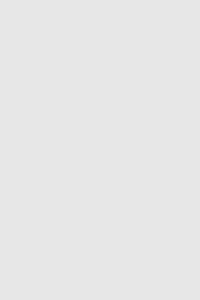
117
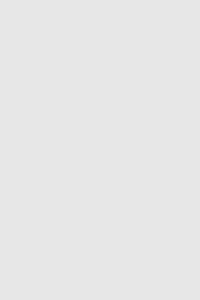
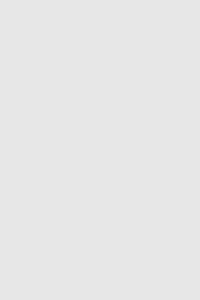
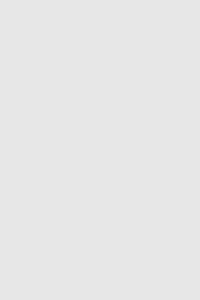
118
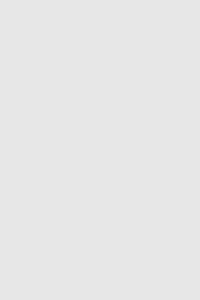
119
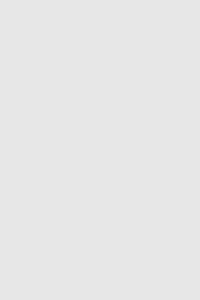
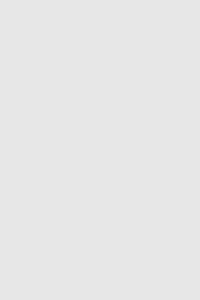
120
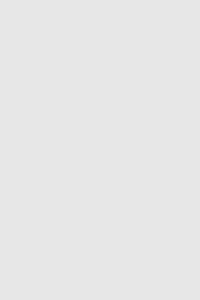
121
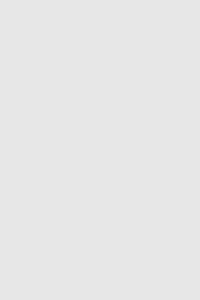
122
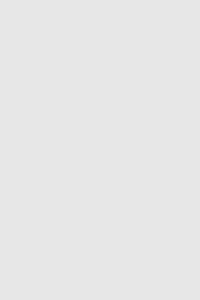
123
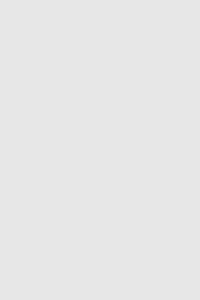
124
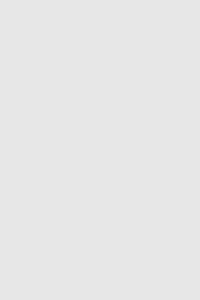
125
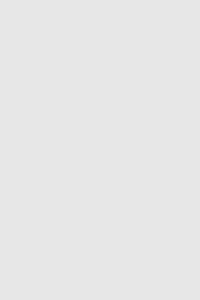
126
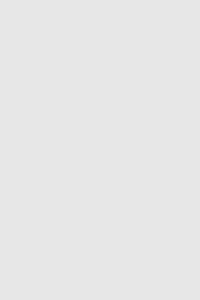
127
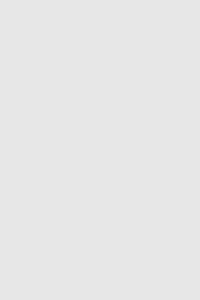
128
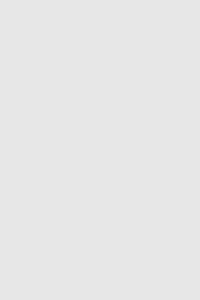
129
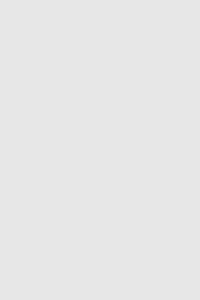
130
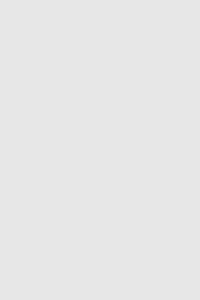
131
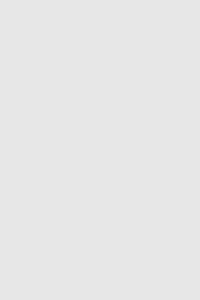
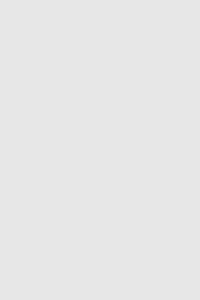
132
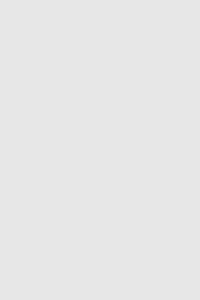
133
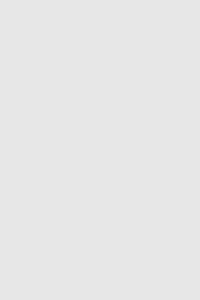
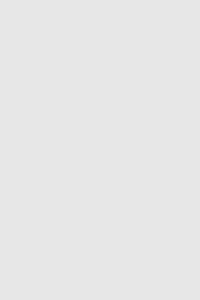
134
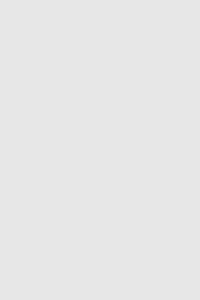
135
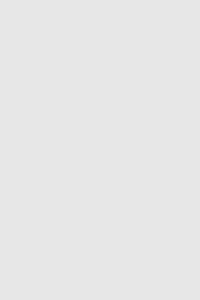
136
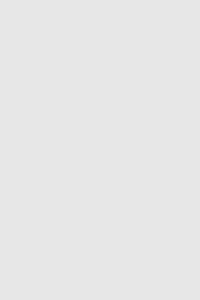
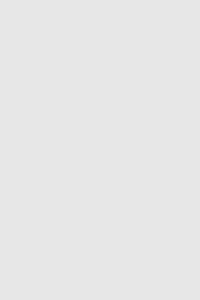
137
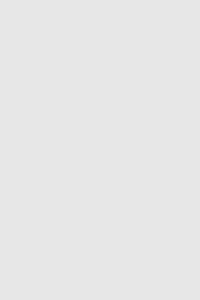
138
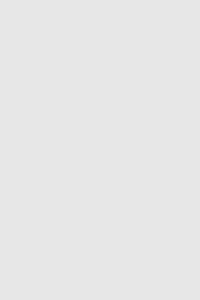
139
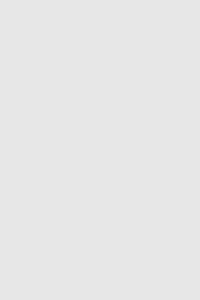
140
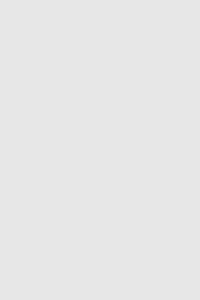
141
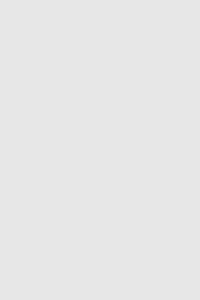
142
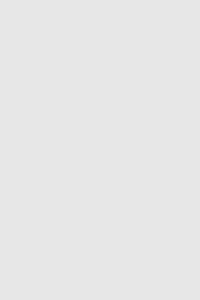
143
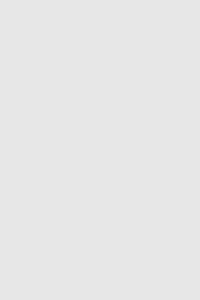
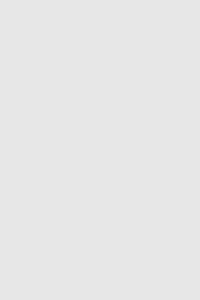
144
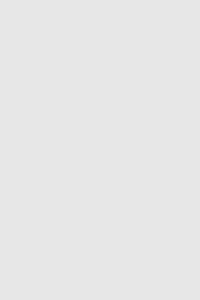
145
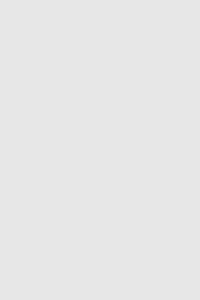
146
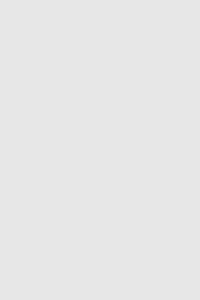
147
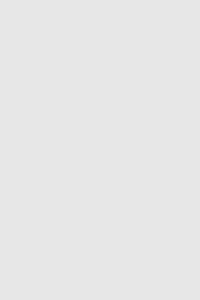
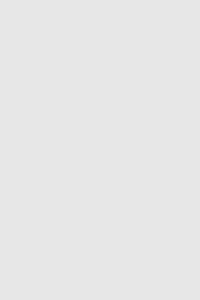
148
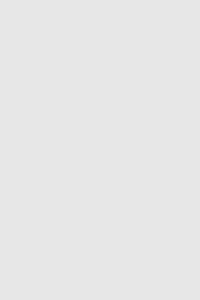
149
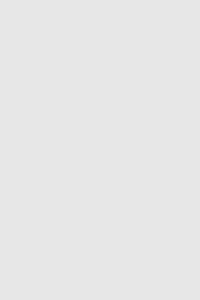
150
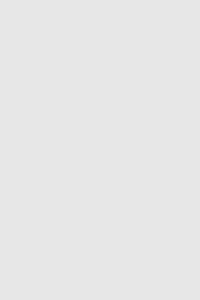
151
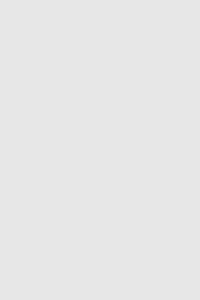
152
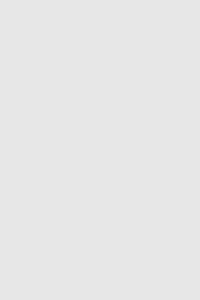
153
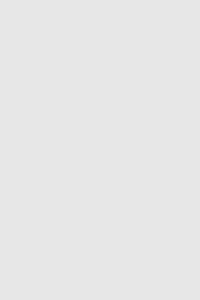
154
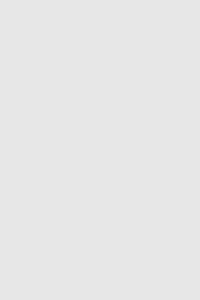
155
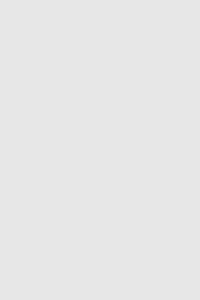
156
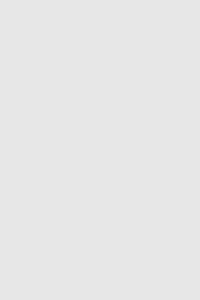
157
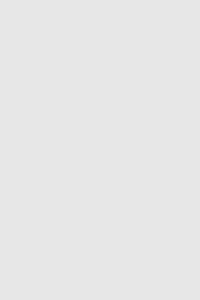
158
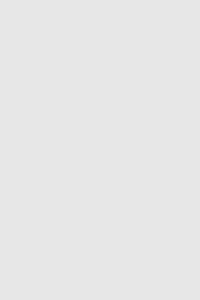
159
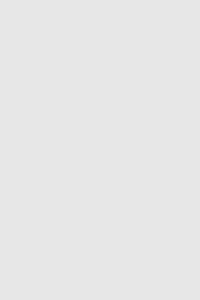
160
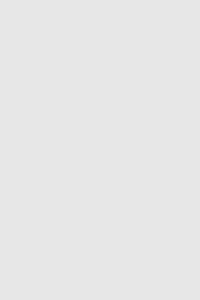
161
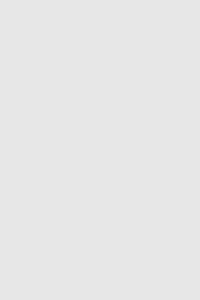
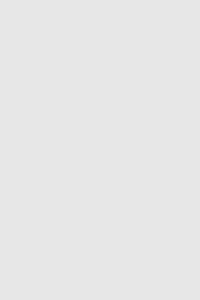
162
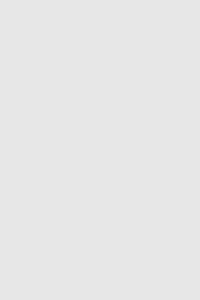
163
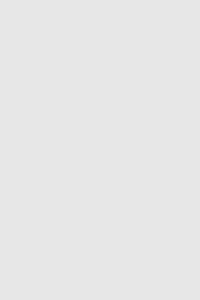
164
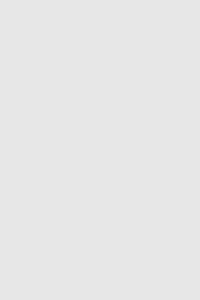
165
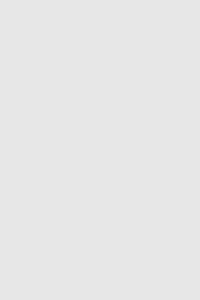
166
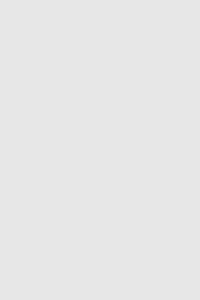
167
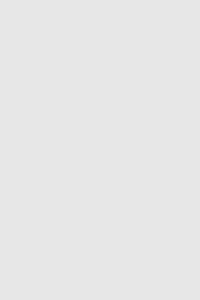
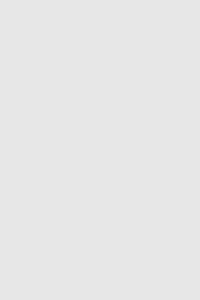
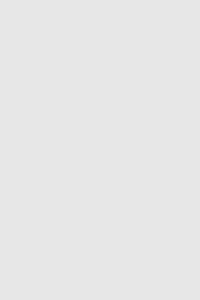
168
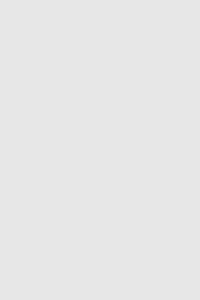
169
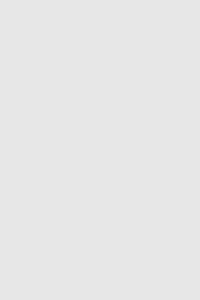
170
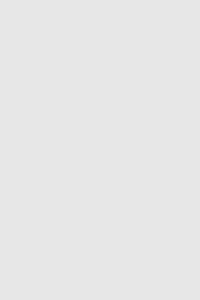
171
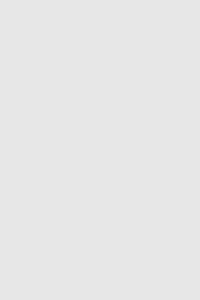
172
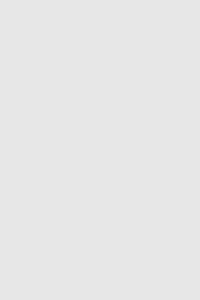
173
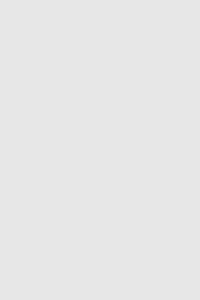
174
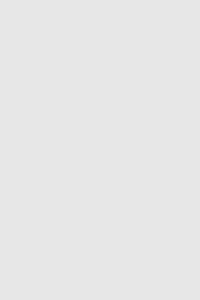
175
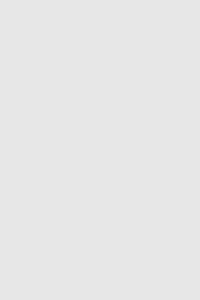
176
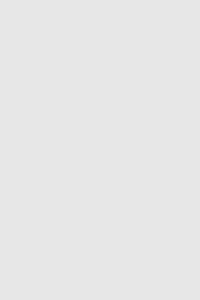
177
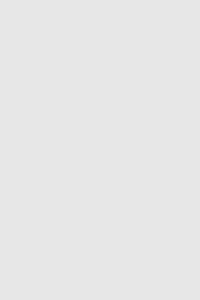
178
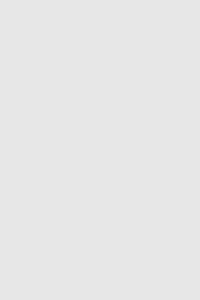
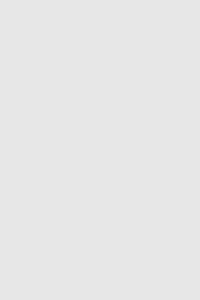
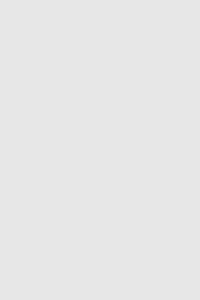
179
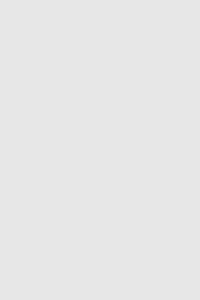
180
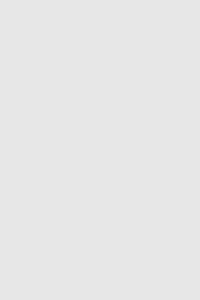
181
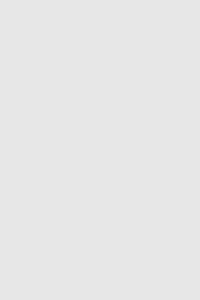
182
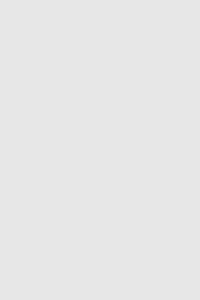
183
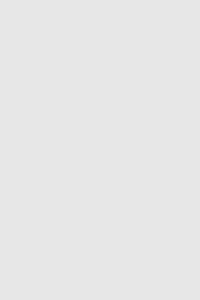
184
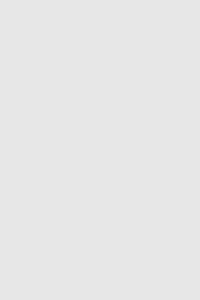
185
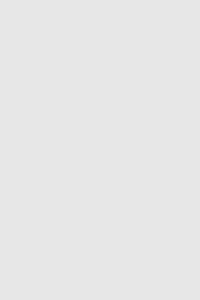
186
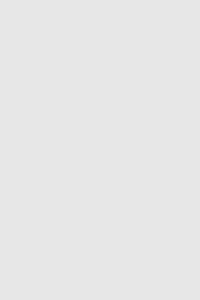
187
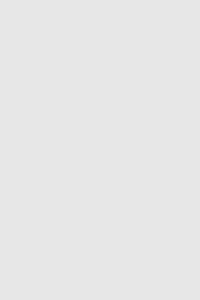
更重要的是SWT_AWT主要是将AWT嵌入SWT中,而ChartComposite则是将chart对象直接转换为SWT中的东东,连右键都是SWT的了~
声明:
永久性关闭本Blog,内容已经迁往 www.lign.name 全新的空间,全新的内容,敬请关注!
posted on 2007-08-21 09:22 阿南 阅读(1079) 评论(1) 编辑 收藏 所属分类: 西安java用户群 、Eclipse-SWT
评论
# re: SWT中使用JFreeChart(无需SWT_AWT)[未登录] 2007-08-21 17:37 寒武纪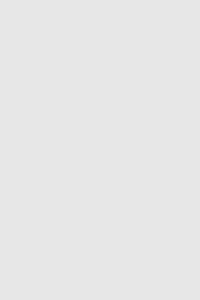